- Published on
Unlocking the Power of the Microsoft Graph SDK: Exploring Different Authentication Methods
- Authors
- Name
- Ivan Gechev
In this post, I will delve into some of the different authentication methods offered by the Microsoft Graph SDK, equipping you with the knowledge and tools necessary to seamlessly integrate your applications with Microsoft services. Get ready to unleash the true potential of the Microsoft Graph SDK and harness the full power of Microsoft 365!
What is Microsoft Graph?
Microsoft Graph is the entry point for data access and management in Microsoft 365. It gives us a unified model that we can use to access tremendous amounts of data.
Microsoft Graph exposes multiple REST APIs and client libraries allowing us easy data access for various Microsoft cloud services. Some of those include emails, calendars, OneDrive, SharePoint, Teams and many more.
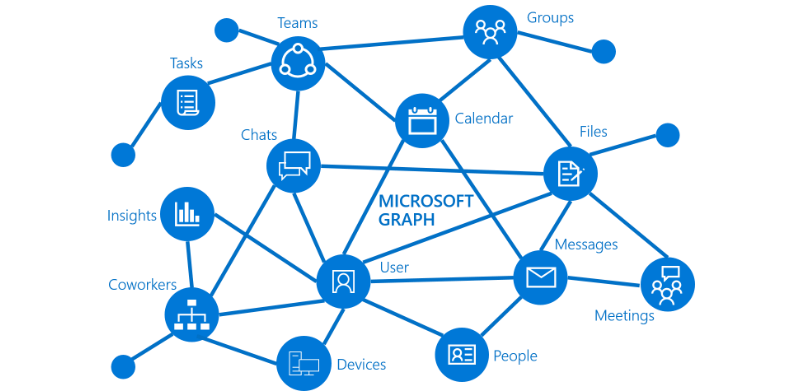
The Microsoft Graph SDK is designed to facilitate the development of high-quality and robust applications that can access and modify data on various Microsoft services. The SDK is available for C#, PowerShell, JavaScript(TypeScript), Java, Go, and PHP. It is also in preview for Python.
In the next sections, we will explore how to authenticate the Microsoft Graph Client in C#.
Installing Microsoft Graph and Setting Things Up
Before we can authenticate, or even use Microsoft Graph in our application, we need to install the package. We also need an additional package that provides the credential models - Azure's Identity package.
We can use the .NET CLI:
dotnet add package Microsoft.Graph
dotnet add package Azure.Identity
Or the Package Manager inside Visual Studio:
Install-Package Microsoft.Graph
Install-Package Azure.Identity
Once this is done, we can move to the next step - setting up our environmental variables:
AZURE_TENANT_ID
- The Azure Active Directory tenant ID.AZURE_CLIENT_ID
- The client or application ID of an App Registration in the tenant.AZURE_CLIENT_SECRET
- A client secret that was generated for the App Registration.AZURE_CLIENT_CERTIFICATE_PATH
- A path to the certificate and private key pair in PEM or PFX format, which can authenticate the App Registration.AZURE_CLIENT_CERTIFICATE_PASSWORD
- Optional - The password protecting the certificate file (only supported for PFX (PKCS12) certificates).AZURE_CLIENT_SEND_CERTIFICATE_CHAIN
- Optional - Specifies whether an authentication request will include an x5c header to support subject name/issuer based authentication.AZURE_USERNAME
- The username of an Azure Active Directory user account.AZURE_PASSWORD
- The password of the Azure Active Directory user account.
Tenant and client IDs are mandatory. Depending on the option you decide to go with, you will need one or more of the other variables.
Microsoft Graph Authentication Using Client Secret
The first authentication method we will explore uses a client secret:
var tenantId = Environment.GetEnvironmentVariable("AZURE_TENANT_ID");
var clientId = Environment.GetEnvironmentVariable("AZURE_CLIENT_ID");
var clientSecret = Environment.GetEnvironmentVariable("AZURE_CLIENT_SECRET");
var clientSecretCredential = new ClientSecretCredential(
tenantId, clientId, clientSecret);
var scopes = new [] { "https://graph.microsoft.com/.default" };
var graphClient = new GraphServiceClient(clientSecretCredential, scopes);
First, we assign the values retrieved from the environment variables AZURE_TENANT_ID
, AZURE_CLIENT_ID
, and AZURE_CLIENT_SECRET
to the tenantId
, clientId
, and clientSecret
variables using the Environment.GetEnvironmentVariable
method.
Then, we create a new clientSecretCredential
variable using the ClientSecretCredential
constructor, with the previously obtained tenant and client IDs, as well as the client secret as parameters.
Next, we initialize a string
array called scopes
with a single element that defines the scope for the authentication context. The scope we use, /.default
, refers to the list of permissions defined on the application registration in Azure.
Finally, we create our Microsoft Graph Client using the GraphServiceClient
constructor, passing the clientSecretCredential
and scopes
as arguments.
Microsoft Graph Authentication Using Client Certificate
The next approach we're going to use utilizes a client certificate:
var tenantId = Environment.GetEnvironmentVariable("AZURE_TENANT_ID");
var clientId = Environment.GetEnvironmentVariable("AZURE_CLIENT_ID");
var certificatePath = Environment.GetEnvironmentVariable("AZURE_CLIENT_CERTIFICATE_PATH");
var clientCertificateCredential = new ClientCertificateCredential(
tenantId, clientId, certificatePath);
var scopes = new [] { "https://graph.microsoft.com/.default" };
var graphClient = new GraphServiceClient(clientCertificateCredential, scopes);
As with the previous approach, first, we assign tenantId
and clientId
. Then we assign the value of AZURE_CLIENT_CERTIFICATE_PATH
to the certificatePath
variable.
Next, we move on to create the clientCertificateCredential
variable using the ClientCertificateCredential
constructor. First, we pass tenantId
and clientId
, as the last parameter we use certificatePath
.
The scopes
initialization is identical to the previous approach. The graphClient
one differs only by the clientCertificateCredential
parameter.
Microsoft Graph Authentication Using Username and Password
With this approach, we can utilize authentication to Azure Active Directory using a user's username and password:
var tenantId = Environment.GetEnvironmentVariable("AZURE_TENANT_ID");
var clientId = Environment.GetEnvironmentVariable("AZURE_CLIENT_ID");
var username = Environment.GetEnvironmentVariable("AZURE_USERNAME");
var password = Environment.GetEnvironmentVariable("AZURE_PASSWORD");
var usernamePasswordCredential = new UsernamePasswordCredential(
username, password, tenantId, clientId);
var scopes = new [] { "https://graph.microsoft.com/.default" };
var graphClient = new GraphServiceClient(usernamePasswordCredential, scopes);
We start by creating and assigning the corresponding environmental variables to the tenantId
, clientId
, username
, and password
variables, respectively using the already familiar Environment.GetEnvironmentVariable
method. After that, we initialize the usernamePasswordCredential
variable using the UsernamePasswordCredential
constructor, with the previously defined variables as parameters.
Last but not least, we create the graphClient
object using the well-known by now constructor, passing the usernamePasswordCredential
and scopes
as arguments. You should be aware that if the user has multi-factor authentication (MFA) enabled this credential flow will fail to acquire a token, ultimately throwing an AuthenticationFailedException
.
IMPORTANT:
This approach does not support accounts that have MFA enabled. This approach is also NOT recommended as it requires an extreme degree of trust and credential exposure. Please use it with the utmost caution and only when other authentication methods are not an option.
Microsoft Graph Authentication Using Environmental Credentials
The final method we will look at is the shortest:
var environmentCredential = new EnvironmentCredential();
var scopes = new [] { "https://graph.microsoft.com/.default" };
var graphClient = new GraphServiceClient(environmentCredential, scopes);
We create a variable called environmentCredential
and initialize it with the parameterless EnvironmentCredential
constructor. After this is done, we initialize the graphClient
, passing the environmentCredential
and the scopes
.
Depending on the environment variables defined, this particular approach will try to authenticate using a ClientSecretCredential
, ClientCertificateCredential
, or UsernamePasswordCredential
. It will use this exact order. You have to be aware that there is a chance to get authenticated using the UsernamePasswordCredential
, which warrants extreme caution.
Conclusion
By exploring authentication using secrets, certificates, usernames and passwords, as well as environmental credentials, you now possess the knowledge and tools necessary to effortlessly integrate your applications with Microsoft services.
The Microsoft Graph SDK, combined with these authentication methods, empowers you to unlock the vast potential of the Microsoft Graph APIs. Whether you're working with emails, calendars, OneDrive, SharePoint, Teams, or any other Microsoft cloud services, the SDK's client libraries in various programming languages offer flexibility and ease of use.
One thing you must remember when implementing authentication is that it is crucial to consider security best practices and choose the appropriate method based on your specific scenario. While client secrets, client certificates, and environmental credentials provide secure options, it's important to exercise extreme caution when using username and password authentication.
With the knowledge gained from this post, you are now equipped to confidently authenticate your applications and access the vast array of data and services through the Microsoft Graph SDK. Embrace the possibilities and elevate your application development with Microsoft 365!